Nuxt.js Contentfulチートシート
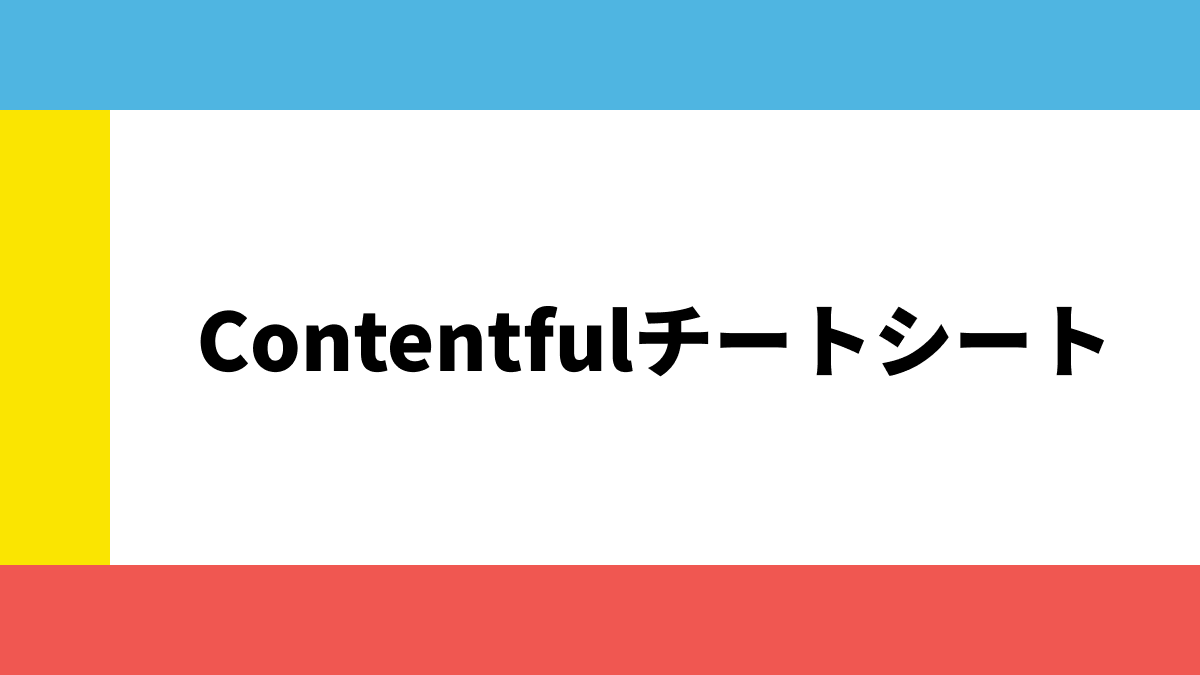
2023.03.27
2023.04.17
公式リファレンスの内容から、Entryの検索について自分なりに日本語で分かりやすくまとめてみました。
目次
- contentfulクライアントのインストール
- クライアントインスタンス生成
- エントリの検索 基本形
- ・getEntry
- ・getEntries
- エントリの検索 オプション
- ・完全一致
- ・部分一致「[match]」
- ・除外「[ne]」
- ・複数完全一致「[all]」
- ・複数一致「[in]」
- ・複数除外「[nin]」
- ・存在チェック「[exists]」
- ・範囲指定「[lt][lte][gt][gte]」
- ・全文検索
- ・取得項目の指定「select」
- ・取得する階層を指定「include」
- ・ソート「order」
- ・取得数指定「limit」
- ・オフセット指定「skip」
- ・位置近接検索「[near]」
- ・位置範囲検索「[within]」
- ・エントリへのリンク「links_to_entry」
- ・アセットへのリンク「links_to_asset」
- ・アセットのMIMEタイプ「mimetype_group」
- ・ロケールの指定「locale」
contentfulクライアントのインストール
まずいつもの方法でインストール
npm i contentful
クライアントインスタンス生成
nuxtプロジェクトで使う際はpluginに追加。
各種ContentfulキートークンはContentful > Settings > API keysを参照。
plugin/contentful.js
const contentful = require('contentful');
/* コンフィグ設定 */
const config =
(process.env.NODE_ENV === "production") ? {
// 本番環境:公開コンテンツを取得
space: コンテントフルのSpace ID,
accessToken: コンテントフルのContent Delivery API - access token,
} : {
// ステージング環境:未公開含めたコンテンツを取得
space: コンテントフルのSpace ID,
accessToken: コンテントフルのContent Preview API - access token,
host: "preview.contentful.com"
};
module.exports = contentful.createClient(config);
nuxt.config.js
plugins: [
"@/plugins/contentful",
],
エントリの検索 基本形
プラグインをインポートして使用します。
import contentful from "@/plugins/contentful"
…
・単一エントリの取得「getEntry」
戻り値はobjectになります。
contentful.getEntry("エントリID").then(entry => {
console.log(entry.fields.title);
console.log(entry.fields.body);
console.log(entry.sys.createdAt);
});
・複数エントリの取得「getEntries」
戻り値は配列になります。
contentful.getEntries({
content_type: "コンテントタイプ",
}).then(entries => {
console.log(entries.items[0].fields.title);
console.log(entries.items[1].fields.title);
console.log(entries.items[2].fields.title);
});
エントリの検索 オプション
いろんなオプションがあるので組み合わせて使ってください。
・「content_type」に指定できるのは1つまでとなります。
・fieldsやsysやmetadataなどの指定は、基本的にContentfulから取得したオブジェクトに含まれている形に沿って指定することができます。(Contentfulから取得したオブジェクトをconsole.logして確認してみてね)
・完全一致
// スラッグ名が「foo」のものを検索
// 配列フィールドに対しても有効です
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug": "foo",
}).then(entries => {
// ~~~
});
// sysの値からももちろん検索可能
contentful.getEntries({
content_type: "コンテントタイプ",
"sys.id": "エントリID",
}).then(entries => {
// ~~~
});
・部分一致「[match]」
// スラッグ名に「foo」という文字列が含まれているものを検索
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug[match]": "foo",
}).then(entries => {
// ~~~
});
・除外「[ne]」
// スラッグ名が「foo」以外のものを検索
// 配列フィールドに対しても有効です
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug[ne]": "foo",
}).then(entries => {
// ~~~
});
・複数完全一致「[all]」
// タグに「foo」と「bar」2つが含まれるものを検索 スペースなしカンマで複数条件を指定すること
contentful.getEntries({
content_type: "コンテントタイプ",
"metadata.tags.sys.id[all]": "foo,bar",
}).then(entries => {
// ~~~
});
・複数一致「[in]」
// スラッグ名が「foo」または「bar」のものを検索 スペースなしカンマで複数条件を指定すること
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug[in]": "foo,bar",
}).then(entries => {
// ~~~
});
・複数除外「[nin]」
// スラッグ名が「foo」と「bar」以外のものを検索 スペースなしカンマで複数条件を指定すること
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug[nin]": "foo,bar",
}).then(entries => {
// ~~~
});
・存在チェック「[exists]」
// スラッグ名が存在する(入力されている)ものを検索
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.slug[exists]": true,
}).then(entries => {
// ~~~
});
・範囲指定「[lt][lte][gt][gte]」
// [lt]: 未満
// [lte]:以下
// [gt]:より大きい
// [gte]: 以上。
// 数値・日付にのみ有効。
// エントリ作成日が2022/01/01以降のものを検索
contentful.getEntries({
content_type: "コンテントタイプ",
"sys.createdAt[gte]": "2022-01-01T00:00:00Z",
}).then(entries => {
// ~~~
});
・全文検索
// エントリ内容に「foo」が含まれるものすべてを検索
contentful.getEntries({
content_type: "コンテントタイプ",
query: "foo",
}).then(entries => {
// ~~~
});
・取得項目を指定「select」
// 「sys.id」と「fields.title」のみを取得
// 深さ2までのプロパティのみを選択可 最大100個
contentful.getEntries({
content_type: "コンテントタイプ",
select: "sys.id,fields.title",
}).then(entries => {
// ~~~
});
・取得する階層を指定「include」
// エントリ内Referencesの階層(子エントリ,孫エントリなど)を指定
contentful.getEntries({
content_type: "コンテントタイプ",
include: 2,
}).then(entries => {
// ~~~
});
・ソート「order」
// ソート対象フィールドタイプ:文字列, 整数, 小数, 日付, 真偽値
// エントリ作成日昇順で検索
contentful.getEntries({
content_type: "コンテントタイプ",
order: "sys.createdAt",
}).then(entries => {
// ~~~
});
// エントリ作成日降順で検索
contentful.getEntries({
content_type: "コンテントタイプ",
order: "-sys.createdAt",
}).then(entries => {
// ~~~
});
// 第二ソート条件も指定可能
contentful.getEntries({
content_type: "コンテントタイプ",
order: "sys.createdAt,sys.updatedAt",
}).then(entries => {
// ~~~
});
・取得数指定「limit」
// 最大1000件まで
// 5件までの結果を取得
contentful.getEntries({
content_type: "コンテントタイプ",
limit: 5,
}).then(entries => {
// ~~~
});
・オフセット指定「skip」
// 10件目からの結果を取得
contentful.getEntries({
content_type: "コンテントタイプ",
skip: 10,
}).then(entries => {
// ~~~
});
「limit」と「skip」を組み合わせてページングを実現できます。
contentful.getEntries({
content_type: "コンテントタイプ",
limit: 20,
skip: 現在ページ数 * 20,
}).then(entries => {
// ~~~
});
・位置近接検索「[near]」
// 緯度30度, 経度-100度に最も近いロケーションを持つエントリを検索
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.location[near]": "30,-100",
}).then(entries => {
// ~~~
});
・位置範囲検索「[within]」
// 緯度30度, 経度-100度から緯130度, 経度-200度までの長方形範囲を検索
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.location[within]": "30,-100,130,-200",
}).then(entries => {
// ~~~
});
// 緯度30度, 経度-100度から半径500kmの円範囲を検索
contentful.getEntries({
content_type: "コンテントタイプ",
"fields.location[within]": "30,-100,500",
}).then(entries => {
// ~~~
});
・エントリへのリンク「links_to_entry」
// "エントリID"へのリンクを持つフィールドの検索
contentful.getEntries({
content_type: "コンテントタイプ",
links_to_entry: "エントリID",
}).then(entries => {
// ~~~
});
・アセットへのリンク「links_to_asset」
// "アセットID"へのリンクを持つフィールドの検索
contentful.getEntries({
content_type: "コンテントタイプ",
links_to_asset: "アセットID",
}).then(entries => {
// ~~~
});
・アセットのMIMEタイプ「mimetype_group」
// 指定可能:attachment, plaintext, image, audio, video, richtext,
// presentation, spreadsheet, pdfdocument, archive, code, markup
// 画像アセットを検索「getAssets」
contentful.getAssets({
mimetype_group: "image",
}).then(entries => {
// ~~~
});
・ロケールの指定「locale」
// ロケールが「en-US」のものを検索
contentful.getEntries({
content_type: "コンテントタイプ",
locale: "en-US",
}).then(entries => {
// ~~~
});